Odoo 17 introduces many powerful features for developers. One is the OWL JS Framework, a modern JavaScript framework that provides a component-based system for building dynamic and interactive user interfaces. Lifecycle hooks in OWL JS play a crucial role in managing the lifecycle of components, enabling developers to handle events and states efficiently. This blog post will help you to understand OWL JS lifecycle hooks in Odoo 17.
Table of Contents
What are OWL JS Lifecycle Hooks in Odoo 17
OWL Js lifecycle hooks are special methods in OWL components that allow developers to perform specific actions during the component’s lifecycle. These hooks can be invoked at specific points during the component’s lifetime. These OWL hooks provide entry points into processes like:
- Initialization: When a component is first being created.
- Mounting: When a component is inserted into the DOM.
- Updating: When changes in props trigger a component to re-render.
- Destruction: When a component is removed from the DOM.
Key Lifecycle Hooks in OWL JS
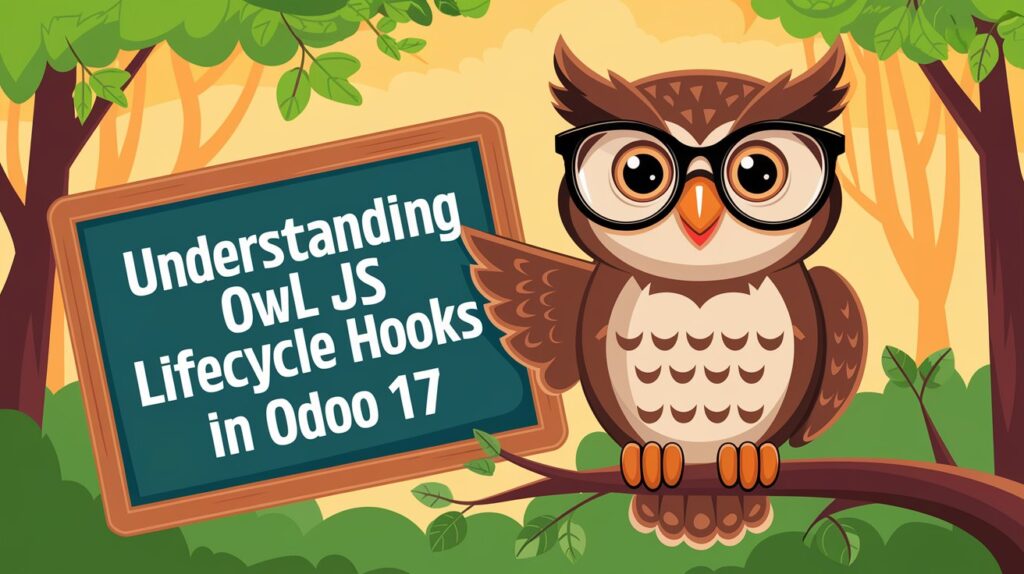
Let’s look at some of the most important OWL lifecycle hooks you will find in Odoo 17:
setup()
Call immediately after the component is created. By enabling developers to provide the initial state, configure event listeners, and create any required connections to other resources, this hook is essential to providing the basis for the component’s lifetime. For example
import { Component } from '@odoo/owl';
class Counter extends Component {
setup() {
this.state = useState({ count: 0 });
}
increment() {
this.state.count++;
}
}
Counter.template = xml`<div>
<button t-on-click="increment">Increment</button>
<p>Count: <t t-esc="state.count"/></p>
</div>`;
JavaScriptonMounted():
This lifecycle hook interacts with the DOM after the first rendering. The mounted hook is called when a component is attached to the DOM. You can interact with the DOM, add event listeners, or call any method at this hook.
The mounted hook allows developers to ensure that a component is fully integrated into the DOM and ready for user interaction. For example,
setup() {
onMounted(() => {
window.addEventListener("scroll", this.handleScroll);
});
}
JavaScriptonWillStart():
The onWillStart() hook is an asynchronous method that is called before the component is rendered for the first time. This is ideal for fetching data or performing asynchronous operations.
By using this hook, you can ensure that the data is available before the components are rendered. This will provide you with a smoother user experience.
setup() {
onWillStart(async () => {
this.data = await fetchData();
});
}
JavaScriptOnWillRender()
This hook onWillRender() allows executing code just before a component is rendered. This hook can be useful for performing actions that need to occur right before rendering, such as manipulating component data. For example,
setup() {
onWillRender(() => {
console.log("Component about to render");
});
}
JavaScriptonWillUpdateProps()
This hook is called when a component receives a new prop. This can be used to react to prop change and update the component’s state.
Before rendering, developers can use the willUpdateProps hook to update the internal state of the component depending on the new props, retrieve data, or make API requests. For instance,
setup() {
onWillUpdateProps(async (nextProps) => {
this.data = await fetchData(nextProps.id);
});
}
JavaScriptonWillUnmount()
This onWillUnmount hook is called before the component is removed from the DOM. It mostly cleans up resources such as event listeners or intervals.
Developers can ensure the component is appropriately cleaned up before being removed from the DOM using the willUnmount hook. Usage example:
setup() {
onMounted(() => {
window.addEventListener("resize", this.handleResize);
});
onWillUnmount(() => {
window.removeEventListener("resize", this.handleResize);
});
}
JavaScriptonWillDestroy()
This hook helps to clean operations for inactive components, which is called when a component is destroyed, regardless of whether it has been mounted or not.
It is useful for releasing resources, clearing timers, or performing any other necessary cleanup tasks. Let’s take an example:
setup() {
onWillDestroy(() => {
// Clean up resources
this.cleanup();
});
}
JavaScriptHandling Errors with onError
Errors may arise within components or their sub-components despite our best efforts. A method to detect and manage these issues is offered via the onError hook.
Developers can apply error-handling logic, such as displaying error messages, logging errors for debugging, or gracefully recovering from problems to ensure a seamless user experience, by using the onError hook. Usage example:
setup() {
onError((error) => {
console.error("Component error:", error);
// Perform error handling logic
});
}
JavaScriptBest Practices for Using OWL Lifecycle Hooks
- Avoid Side Effects in
Setup
: Use Setup for initializing state and properties. Perform side effects like API calls in onWIllStart or onMounted. - Cleanup Resources in
willUnmount
: Always clean up event listeners, intervals, or other resourceswillUnmount
to prevent memory leaks. - Minimize Work in Hooks: Keep the logic in hooks concise to maintain readability and performance.
- Leverage Reactive State: Use
useState
to manage reactive state, ensuring updates trigger re-renders efficiently.
Related Post:
>> What is Odoo OWL JS Framework? An Odoo’s Front-End Framework
>> Odoo.sh VS Odoo Online VS Odoo On-Premises
Conclusion
Lifecycle hooks in Owl provide deep control over component behavior in Odoo 17. Understanding these hooks allows you to create powerful and efficient apps while assuring clean and maintainable code. Experiment with these hooks in your projects to learn how to use them properly and maximize Owl’s possibilities.
This comprehensive guide to understanding OWL JS lifecycle hooks in Odoo 17 teaches you how to use Owl’s lifecycle system and create robust and efficient components.
Thank You and Happy Coding!