Creating a registration and login form using PHP and MySQL is essential for building dynamic and secure web applications. Whether you are working on a blog, an e-commerce site, or a user-driven platform, allowing users to register and log in ensures personalized experiences and data management. In this guide, you will learn how to build a fully functional system that securely manages user data and enables seamless authentication. This step-by-step tutorial simplifies the process, making it easy for developers to integrate into their projects.
Table of Contents
Steps to Create Registration and Login Form Using PHP and MySQL
Set Up Your Database
To begin, you need to create a database to store user details. First, you need to set up and install WAMP or XAMPP. You can use tools like WAMP or XAMPP servers for this purpose. These servers provide a local development environment for running PHP and MySQL.
Create a Project Folder
Next, navigate to the www
folder if you’re using WAMP, or the htdocs
folder if you’re using XAMPP. Inside this directory, create a new folder for your project. You can name this folder anything you like, such as user_auth
.
Create the Required Files
Within the project folder, create the following files:
registration.php
: This file will handle the user registration process.login.php
: This file will handle the user login process.
You can choose custom names for these files, but ensure they are meaningful and reflect their purpose.
Start Writing the Code
With the basic setup complete, you can now start writing the PHP and MySQL code to handle user registration and login functionality.
PHP Code For Registration Form:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Registration Form</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.form-container {
background: #fff;
padding: 20px 30px;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 400px;
width: 100%;
}
.form-container h1 {
text-align: center;
margin-bottom: 20px;
color: #333;
}
.form-container label {
display: block;
margin-bottom: 10px;
font-weight: bold;
color: #555;
}
.form-container input[type="text"],
.form-container input[type="email"],
.form-container input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ddd;
border-radius: 5px;
font-size: 16px;
color: #333;
background-color: #f9f9f9;
}
.form-container input[type="text"]:focus,
.form-container input[type="email"]:focus,
.form-container input[type="password"]:focus {
border-color: #007bff;
outline: none;
}
.form-container input[type="submit"] {
width: 100%;
padding: 10px;
background-color: #007bff;
color: #fff;
font-size: 16px;
font-weight: bold;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.form-container input[type="submit"]:hover {
background-color: #0056b3;
}
.form-container a {
color: #007bff;
text-decoration: none;
font-weight: bold;
}
.form-container a:hover {
text-decoration: underline;
}
.form-container p {
text-align: center;
margin-top: 20px;
font-size: 14px;
color: #555;
}
.error-message {
color: red;
font-size: 14px;
margin-bottom: 15px;
}
.success-message {
color: green;
font-size: 14px;
margin-bottom: 15px;
}
</style>
</head>
<body>
<div class="form-container">
<h1>Sign Up</h1>
<form method="post" action="">
<p>Already registered? <a href="login.php" title="login">Log in</a></p>
<label for="username">Username*</label>
<input type="text" id="username" name="username" required>
<label for="email">Email*</label>
<input type="email" id="email" name="email" required>
<label for="password">Password*</label>
<input type="password" id="password" name="password" required>
<label for="confirm_password">Confirm Password*</label>
<input type="password" id="confirm_password" name="confirm_password" required>
<input type="submit" value="Sign Up" name="sign_up">
</form>
</div>
</body>
</html>
<?php
$conn = new mysqli("localhost", "root", "", "registration");
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if (isset($_POST['sign_up'])) {
$username = $_POST['username'];
$email = $_POST['email'];
$password = $_POST['password'];
$confirm_password = $_POST['confirm_password'];
if ($password !== $confirm_password) {
echo "<p class='error-message'>Error: Passwords do not match. Please try again.</p>";
} else {
$query = $conn->prepare("SELECT * FROM user_data WHERE username = ? OR email = ?");
$query->bind_param("ss", $username, $email);
$query->execute();
$result = $query->get_result();
if ($result->num_rows > 0) {
echo "<p class='error-message'>Error: Username or email already exists. Please choose a different one.</p>";
} else {
$sql = $conn->prepare("INSERT INTO user_data (username, password, email) VALUES (?, ?, ?)");
$sql->bind_param("sss", $username, $password, $email);
if ($sql->execute()) {
echo "<p class='success-message'>You have successfully signed up.</p>";
} else {
echo "<p class='error-message'>Error: " . $conn->error . "</p>";
}
}
$query->close();
}
}
$conn->close();
?>
PHP
Please use this code in the registration.php File.
Code Explanation:
Frontend (HTML + CSS):
- HTML Structure:
- A simple registration form is created with fields for username, email, password, and confirm password.
- A submit button (
Sign Up
) sends form data via POST to the same script for processing. - Includes a link to the login page for existing users.
- CSS Styling:
- The form is styled for a clean and responsive layout.
- Focus effects and hover states are added for interactivity (e.g., button hover, input focus).
Backend (PHP):
Database Connection:
- A MySQL connection is established using
new mysqli()
with parameters: host (localhost
), username (root
), password (empty), and database name (registration
). - Connection errors are handled using
connect_error
.
Form Submission Handling:
- Form data is submitted using the
POST
method when the “Sign Up” button is clicked.
Password Matching:
- Check if
password
andconfirm_password
fields match. - If they don’t, display an error message.
Check for Existing Users:
- Query the database (
user_data
table) to see if the username or email already exists using a prepared statement:
$query = $conn->prepare("SELECT * FROM user_data WHERE username = ? OR email = ?");
PHP- If a record is found, display an error message.
Insert New User:
- If the user does not exist, insert the new user data into the database using a prepared statement:
$sql = $conn->prepare("INSERT INTO user_data (username, password, email) VALUES (?, ?, ?)");
PHP- Use
bind_param()
to securely insert user data into the query.
Feedback:
- If the insertion is successful, display a success message.
- If an error occurs during insertion, display an error message with the error details.
PHP Code FOR Login Form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Login</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f4f4f9;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.form-container {
background: #fff;
padding: 20px 30px;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
max-width: 400px;
width: 100%;
}
.form-container h1 {
text-align: center;
margin-bottom: 20px;
color: #333;
}
.form-container label {
display: block;
margin-bottom: 10px;
font-weight: bold;
color: #555;
}
.form-container input[type="email"],
.form-container input[type="password"] {
width: 100%;
padding: 10px;
margin-bottom: 15px;
border: 1px solid #ddd;
border-radius: 5px;
font-size: 16px;
color: #333;
background-color: #f9f9f9;
}
.form-container input[type="email"]:focus,
.form-container input[type="password"]:focus {
border-color: #007bff;
outline: none;
}
.form-container input[type="submit"] {
width: 100%;
padding: 10px;
background-color: #007bff;
color: #fff;
font-size: 16px;
font-weight: bold;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.form-container input[type="submit"]:hover {
background-color: #0056b3;
}
.form-container a {
color: #007bff;
text-decoration: none;
font-weight: bold;
}
.form-container a:hover {
text-decoration: underline;
}
.form-container p {
text-align: center;
margin-top: 20px;
font-size: 14px;
color: #555;
}
.error-message {
color: red;
font-size: 14px;
margin-bottom: 15px;
}
.success-message {
color: green;
font-size: 14px;
margin-bottom: 15px;
}
</style>
</head>
<body>
<div class="form-container">
<h1>Login</h1>
<form method="post">
<p>Not registered yet? <a href="registration.php" title="registration">Sign Up!</a></p>
<label for="log_email">Email*</label>
<input type="email" id="log_email" name="log_email" required>
<label for="log_password">Password*</label>
<input type="password" id="log_password" name="log_password" required>
<input type="submit" value="Log in" name="login">
</form>
</div>
</body>
</html>
<?php
$conn = new mysqli("localhost", "root", "", "registration");
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
if (isset($_POST['login'])) {
$email = $_POST['log_email'];
$pswd = $_POST['log_password'];
$sql = $conn->prepare("SELECT * FROM user_data WHERE email = ? AND password = ?");
$sql->bind_param("ss", $email, $pswd);
$sql->execute();
$result = $sql->get_result();
if ($result->num_rows > 0) {
echo "<p class='success-message'>Welcome! Redirecting to Home Page...</p>";
} else {
echo "<p class='error-message'>Error: Incorrect email or password. Please try again!</p>";
}
}
?>
PHPCode Explanation:
HTML + CSS: Creates a styled login form with email and password fields, plus a link to the registration page.
PHP Backend:
- Connects to the MySQL database (
registration
). - On form submission, check if the entered email and password match a record in the
user_data
table using a prepared statement. - Shows a success message if the credentials are correct; otherwise, shows an error message.
Output of Registration and Login Form using PHP and MySQL
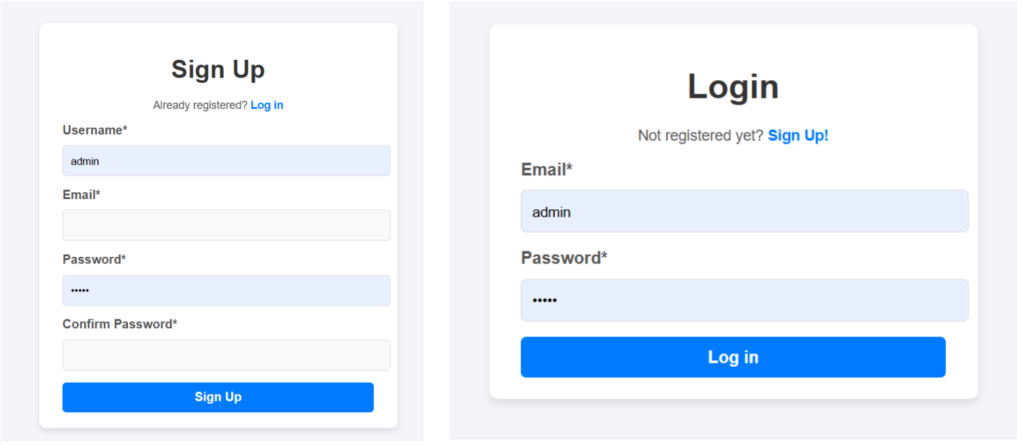
Related Posts >>
>>How to Get Checkbox Value in JQuery
>>How to Create Dynamic HTML Table Using JavaScript
Conclusion: Registration and Login Form using PHP and MySQL
Building a registration and login form using PHP and MySQL involves connecting to a database, validating user inputs, and verifying credentials securely. This implementation provides a clear structure for handling user authentication while displaying appropriate messages for success or failure. Ensure to keep the database and code optimized for efficient handling of user data, making your application more reliable and user-friendly.
Happy Coding!