Sorting arrays is the fundamental concept of any programming language, especially when managing data efficiently. In PHP, several built-in functions help to manage the sorting of arrays. There are times when understanding and implementing custom sorting algorithms can be valuable. Writing custom code for sorting arrays allows you to optimize code for specific use cases where on the other hand built-in functions may not be ideal for it. In this blog post, we will explore 5 ways to sort array in PHP Without using inbuilt function.
In this blog post, we will start with classic algorithms like Bubble Sort, Selection Sort, and Insertion Sort, to more unique approaches using functions like “min()”, “current()”, and “next()”, I’ll write all 5 ways to sort array in PHP without using inbuilt function with the proper explanation that helps you to understand each line of code. It will help you improve your coding skills and will help to prepare for an interview. Let; ‘s dive into the different ways to sort array in PHP without using inbuilt function and how they can be implemented step-by-step.
5 ways to Sort Array in PHP Without Using Inbuilt Function
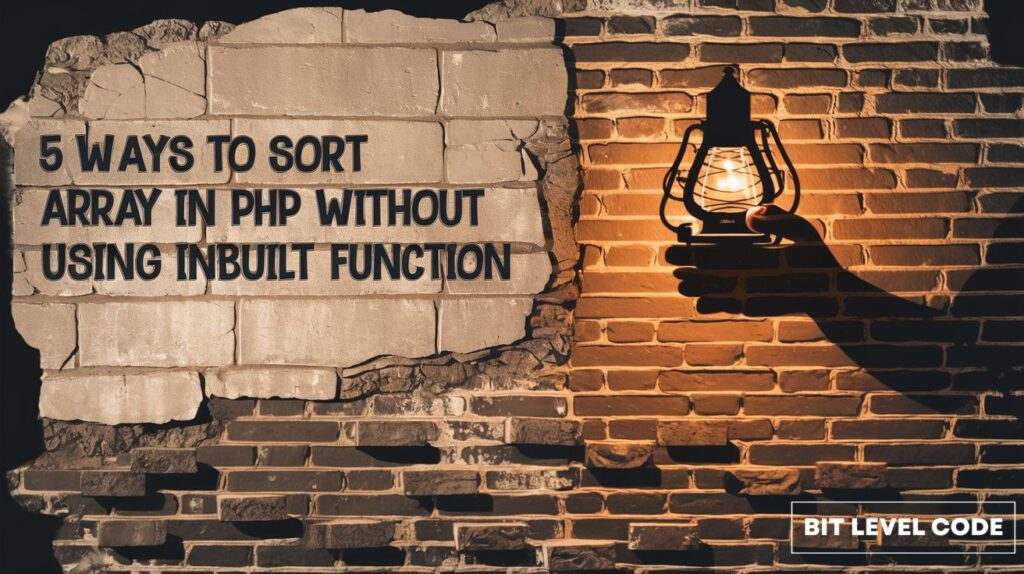
Here are the 5 ways to sort array in PHP without using inbuilt function:
- Sort an Array using Bubble Sort
- Sort an Array using Selection Sort
- Sort an Array using Insertion Sort
- Sort an Array using the min() function
- Sort an Array using the current() and next() function
Sort an Array using Bubble Sort:
Bubble sort is a comparison-based algorithm. During the bubble sort process, we compared each element to its adjacent and sorted them if the comparison revealed that the order was incorrect.
<?php
function bubbleSort(&$array) {
$len = count($array);
for ($i = 0; $i < $len - 1; $i++) {
for ($j = 0; $j < $len - $i - 1; $j++) {
if ($array[$j] > $array[$j + 1]) {
$temp = $array[$j];
$array[$j] = $array[$j + 1];
$array[$j + 1] = $temp;
}
}
}
}
$array = [5,4,3,2,1];
bubbleSort($array);
echo "Sorted array: \n";
print_r($array);
?>
PHPOutput:
Sorted array:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
)
Sort Array in PHP Without Using Inbuilt FunctionSort an Array using Selection Sort:
Selection sort is all about organizing an array by repeatedly finding the minimum element from the unsorted portion of the array and placing it at the beginning.
<?php
function selectionSort(&$array) {
$len = count($array);
for ($i = 0; $i < $len - 1; $i++) {
$minVal = $i;
for ($j = $i + 1; $j < $len; $j++) {
if ($array[$j] < $array[$minVal]) {
$minVal = $j;
}
}
$temp = $array[$minVal];
$array[$minVal] = $array[$i];
$array[$i] = $temp;
}
}
$array = [5,4,3,2,1];
selectionSort($array);
echo "Sorted array: \n";
print_r($array);
?>
PHPOutput:
Sorted array:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
)
Sort Array in PHP Without Using Inbuilt FunctionSort an Array using Insertion Sort:
Insertion sort is an easy-to-understand and effective sorting method, particularly useful for smaller datasets. The algorithm sorts the array by gradually creating a sorted section, handling one element at a time. The process involves iterating through each unsorted element in the array and inserting it into its correct position within the already sorted portion. This is repeated until the whole array becomes sorted.
<?php
function insertionSort(&$array) {
$len = count($array);
for ($i = 1; $i < $len; $i++) {
$key = $array[$i];
$j = $i - 1;
while ($j >= 0 && $array[$j] > $key) {
$array[$j + 1] = $array[$j];
$j--;
}
$array[$j + 1] = $key;
}
}
$array = [5,4,3,2,1];
insertionSort($array);
echo "Sorted array: \n";
print_r($array);
?>
PHPOutput:
Sorted array:
Array
(
[0] => 1
[1] => 2
[2] => 3
[3] => 4
[4] => 5
)
OutputSort an Array using the min() function:
Firstly, we start a loop to extract the minimum number from the array, then find its index and remove it using array_splice(). This removed element is stored in an empty array. By repeating this process, we can build a sorted array.
<?php
$arr = [10,9,8,7,6,5,4,3,2,1,0,-1,-2,-3,-4,-5,-6,-7,-8,-9,-10];
$sorted = [];
while (count($arr) > 0) {
$min = min($arr);
$min_index = array_search($min, $arr);
$sorted[] = array_splice($arr, $min_index, 1)[0];
}
print_r($sorted);
?>
PHPOutput
Array
(
[0] => -10
[1] => -9
[2] => -8
[3] => -7
[4] => -6
[5] => -5
[6] => -4
[7] => -3
[8] => -2
[9] => -1
[10] => 0
[11] => 1
[12] => 2
[13] => 3
[14] => 4
[15] => 5
[16] => 6
[17] => 7
[18] => 8
[19] => 9
[20] => 10
)
OutputSort an Array using the current() and next() Function:
Firstly, we start a loop and then use the reset function to reset the array, fetching the current value and storing it in “currentIndex” while pointing to the first value of the array. We then check if the value needs to be changed and, if so, swap it. The process will repeat until the array becomes fully sorted.
<?php
$a = array(5, 4, 0,8, 2, 1);
$len = count($a);
for ($i = 0; $i < $len - 1; $i++) {
reset($a);
for ($j = 0; $j < $len - $i - 1; $j++) {
$currentIndex = key($a);
$current = current($a);
$next = next($a);
if ($current > $next) {
$a[$currentIndex] = $next;
$a[key($a)] = $current;
}
}
}
echo "Sorted array: ";
print_r($a);
?>
PHPOutput:
Sorted array: Array
(
[0] => 0
[1] => 1
[2] => 2
[3] => 4
[4] => 5
[5] => 8
)
sort array in php without using functionRelated Post:
>> How to Create JQuery Accordion with Multiple Divs
Conclusion
Sort Array in PHP Without Using Inbuilt Function may seem like a challenging task at first, but as we have to practice and explore, there are lots of ways to do this task with simple algorithms. By implementing algorithms such as Bubble Sort, Selection Sort, Insertion Sort, and even more unique ways to sort an array using ‘min()’, ‘current()’, and next() functions.
These custom sorting techniques not only sharpen your problem-solving skills but also make you a more versatile coder. Whether you are preparing for a coding interview, optimizing performance, or simply want to expand your knowledge, mastering these algorithms is a worthwhile investment.
If any query occurs regarding the sort array in PHP without using inbuilt function feel free to contact us!
Happy Coding!