Are you struggling with jQuery accordion for multiple divs? Using accordion design you can make your content compact and organized. Adding an accordion design will optimize the space and make it easier for users to find and interact with the content they need. We’ll cover step-by-step instructions for how to create a JQuery Accordion with multiple divs
HTML Code for Jquery Accordion With Multiple Divs
To start, you’ll need to create a basic HTML file that sets up the primary structure for your accordion design. Make sure to save your file with the .html
extension so it can be correctly viewed and rendered in a web browser. After setting up the file, copy and paste the following HTML code into it:
This way, you’re providing clear instructions while making it unique.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script>
<link href="faq_style.css" rel="stylesheet">
<script type="text/javascript" src="faq_js.js"></script>
<title>Jquery Accordion With Multiple Divs Tags</title>
</head>
<body>
<div class="container mt-5">
<div class="accordian">
<div class="row">
<div class="col-sm-12">
<div class="accordian-header">
<h1 class="header">Jquery Accordion With Multiple Divs Tags</h1>
</div>
</div>
</div>
<div class="accordian-box">
<div class="row">
<div class="col-sm-12">
<div class="acc-ques">
<strong>Q1:</strong>
What is HTML?
</div>
<div class="acc-ans">
<p class="content">
<strong>Ans:</strong> HTML is the standard markup language for creating Web pages.
Learn what HTML stands for, how it describes the structure of a Web page,
and how to write HTML elements and documents.
</p>
</div>
</div>
</div>
</div>
<div class="accordian-box">
<div class="row">
<div class="col-sm-12">
<div class="acc-ques">
<strong>Q2:</strong>
What is CSS?
</div>
<div class="acc-ans">
<p class="content">
<strong>Ans:</strong> CSS stands for Cascading Style Sheets
CSS describes how HTML elements are to be displayed on screen, paper, or in other media
CSS saves a lot of work. It can control the layout of multiple web pages all at once
External stylesheets are stored in CSS files.
</p>
</div>
</div>
</div>
</div>
<div class="accordian-box">
<div class="row">
<div class="col-sm-12">
<div class="acc-ques">
<strong>Q3:</strong>
What is Javascript?
</div>
<div class="acc-ans">
<p class="content">
<strong>Ans:</strong> JavaScript is the Programming Language for the Web.
JavaScript can update and change both HTML and CSS.
JavaScript can calculate, manipulate and validate data.
</p>
</div>
</div>
</div>
</div>
<div class="accordian-box">
<div class="row">
<div class="col-sm-12">
<div class="acc-ques">
<strong>Q4:</strong>
What is Jquery?
</div>
<div class="acc-ans">
<p class="content">
<strong>Ans:</strong> jQuery is a JavaScript Library.
jQuery greatly simplifies JavaScript programming.
jQuery is easy to learn. Jquery Accordion With Multiple Divs
</p>
</div>
</div>
</div>
</div>
</div>
</div>
</body>
</html>
HTMLCode Explanation of HTML
The <!DOCTYPE html>
the declaration specifies the document type and the version of HTML being used in your webpage, helping the browser understand how to render the content.
- `<html lang = ‘eg’>`: This tag defines the root of the HTML document. The “lang” attribute specifies the language of the element’s content. It’s a good practice to add “lang” attribute for the search engine purpose.
- `<head>`: This section contains metadata and links to include resources like CSS, Javascript, and font-awesome files:
- `<meta charset=”UTF-8″>`: it specifies the character encoding for the document to UTF-8. It will make sure your content will be displayed correctly across different devices and browsers.
- `<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>`: it makes your site responsive on various screen sizes.
- `<link href=”https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css” rel=”stylesheet”>: This link includes the Bootstrap CSS . Do not forget to add this link in your head part. This will help to make your website responsive and attractive.
- `<script src=”https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js”></script>`: This script tag imports jQuery.It’s essential for enhancing interactive elements on your page. Make sure this is added to your head tag.
- · <link href=”faq_style.css” rel=”stylesheet”>: it is a custom CSS file (faq_style.css) where you can add style according to your requirements.
- · <script type=”text/javascript” src=”faq_js.js”></script>: This script tag links to a custom JavaScript file (faq_js.js) . It helps you to add functionalities, and events according to your requirements.
- · <title>Jquery Accordion With Multiple Divs</title>: The title tag is important for SEO(Search Engine Optimization) and it defines the name of the webpage. It appears in the browser’s title bar or tab.
- `<body>`: It contains main the structure of the page.
- `<div class= ”container mt-5”>`: The <div> tag acts like a container which is used to group and style elements. And the class “Container” is a pre-defined class of the bootstrap that helps you to make a container so, you do not need to add any padding or margin to adjust the content of your page. “mt-5” means margin-top will add a margin from the top, it is also a pre-defined function of bootstrap. Here, 5 represents the size of the margin from the top.
- `<div class= ”accordion”>`: This is to give a background color for the container.
- `<div class= “row”>`: The row class is a pre-defined class of bootstrap. It is used to create a horizontal group of columns within the grid system.
- `<div class= “col-sm-12”>`: it’s a pre-defined class of bootstrap that defines a column that specifies the column will occupy 12 columns means the entire width of the row on all types of devices.
- `<div class= “accordion-header”>`: we have used this to make an attractive header for the content. It can be like “Frequently Asked Questions”.
- `<h1 class= “header”>:` We use the h1 tag for the heading tag but most importantly it emphasizes its importance and helps with SEO.
- ` <div class= “accordion-box”>`: Main container for the accordion item. It wraps the question and answers both.
- `<div class= “acc-ques”>`: Holds the question, we have created this section to style our question according to the user’s requirement.
- <strong>Q1:</strong>: this tag is used to emphasize the importance of the text and also helps to bold the text.
- `<div class= “acc-ans”>`: It is because of the answer part so we can easily add the custom CSS depending on the user’s requirement.
- `<p class=”content”>:` p tag is used when you want to add a paragraph it will help you to add a paragraph in a proper format.
- ` ` this used to give a space between the element or the tag. It will add a gap.
- Closing tag: Make sure to close all of the tags like </p>,</div>,</body>,</html>. The closing tag is used to ensure the end of the section.
CSS Code for Jquery Accordion With Multiple Divs
* {
box-sizing: border-box;
}
.accordian {
background-color: aliceblue;
}
.accordian .accordian-header .header {
font-size: 20px;
background: #18202a;
text-align: center;
padding: 20px;
color: #fff;
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
font-weight: bold;
border-radius: 5px 5px 0px 0px;
}
.accordian .accordian-header .header:hover {
background: #000000;
color: #fff;
transition: all 0.5s ease;
}
.accordian-box {
border-bottom: 1px solid rgb(90, 90, 90);
box-shadow: 0 2px 4px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
}
.accordian-box .acc-ques {
padding: 20px;
background: #18202a;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
font-size: 20px;
font-family: 'Times New Roman', Times, serif;
font-weight: bold;
color: #fff;
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
cursor: pointer;
}
.accordian-box .acc-ques strong {
background: #fff;
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
color: rgb(0, 0, 0);
padding: 5px;
border-radius: 5px;
text-align: center;
font-size: 15px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19);
}
.accordian-box .acc-ans .content {
padding: 20px;
font-size: 20px;
font-family: 'Gill Sans', 'Gill Sans MT', Calibri, 'Trebuchet MS', sans-serif;
}
.accordian-box .acc-ans {
display: none;
background-color: aliceblue;
}
Code Explanation of CSS
We’ll enhance the design by adding some CSS to make it visually appealing. To create a CSS file with a .css
extension and ensure it is plagiarism-free, you can follow these steps:
- `* { } `:
- The “*” selector targets all elements on the page.
- The box-sizing property defines how the width and height of an element are calculated.
- .accordian{ }:
- Background-color: The property will add a background color for the container.
- .accordian .accordian-header .header { }:
- This is targeting the heading part. We will add style to the heading.
- Font size: this property will add a font size to your header.
- Background: It will add a background color to your header.
- Text-align: center: it will assign the text to the center position.
- Padding: it will add padding to your text.
- Color: it will change the color of the front.
- Font-family: it allows you to choose the text’s style. Adding this you can add a font style.
- Font-weight: it allows you to add weight to the font.
- Border-radius: The border-radius property is used to create rounded corners on an element.
- .accordian .accordian-header .header: hover{ }: It will target when you hover by mouse or when the mouse pointer is entered.
- Transition: Allows you to create a smooth animation. Ease defines the transition as starting slowly, then speeding up, and then slowing down again.
- Background color and color properties are used to change the background color of the header the and color of the text when the header part is covered by the mouse.
- .accordian-box { }: it indicates the question and answer part both.
- Border-bottom: this proper will add a border at the end of every section.
- Box-shadow: it will add a shadow for each block.
- .accordian-box .acc-ques { }: this part indicates the question part. So We can add style easily according to our requirements.
- Cursor: This pointer changes the mouse pointer to a hand and it indicates this section is clickable.
- I have explained other properties in the above section. You can check that.
- .accordian-box .acc-ques strong { }: This section indicates the Question number.
- .accordian-box .acc-ques .content { }: this section indicates that the content means answers to the questions. We have created this section to add some styling to the content.
- .accordian-box .acc-ques { }: this section indicates the whole answer section.
- Display: the “none” property is used to hide the content by default. But when the user wants to view the content will be visible using jQuery. We will discuss the jQuery in the next section.
JQuery Code for Jquery Accordion With Multiple Divs
Well, let’s move to the next most important part. To implement the functionality of an accordion, jQuery can be used effectively. The accordion effect allows users to toggle between hiding and showing content sections. Here’s how you can implement it:
$(document).ready(function(){
$(".accordian-box").first().find(".acc-ans").slideDown(); // Intially open the first section
$(".accordian-box").on('click',function(){
$(this).find(".acc-ans").slideToggle(); // section can be slide up and down
$(this).siblings().find(".acc-ans").slideUp(); //other section will be slide up
});
});
JqueryJQuery Code Explanation
- (document).ready(function(){ … }); :
- This ensures that the jQuery code inside the function runs only after the entire HTML document has been loaded and is ready to be manipulated.
- $(“.accordian-box”).first().find(“.acc-ans”).slideDown();: We have used this line to open the first section initially.
- $(“.accordian-box”).first(): We have used the first method to select the first section of .accordian-box.
- .find(“.acc-ans”): We used the find method to search the class with .acc-ans. There can be multiple div classes so, to find the particular class name “.acc-ans” we will use the find method.
- .slideDown();: SlideDown function is used to slide the specific section This line of code ensures that the first accordion section is open by default when the page loads.
- $(“.accordian-box”).on(‘click’,function(){ }); : The click event is used to perform any functionality. When any element with the class .accordian-box will be clicked then inside functionality will be performed.
- $(this).find(“.acc-ans”).slideToggle();: when .accordian-box will be clicked then I will find the child element class with “.acc-ans”.SlideToggle is used to slide up and down. If the content is hidden then it will help to slide it down to show the content. If the content is visible it will help you to slide it up. It can perform both slides up and down.
- $(this).siblings().find(“.acc-ans”).slideUp();: It will select all the other siblings of .accordian-box. We used this sibling method to slide up the content. If we click on any “.accordian-box” class it will show only its child content and if any other class with “.accordian-box” is opened it will slideUp the other’s content using the slideUp function. slideUp function helps you to again invisible the content.
Output for JQuery Accordion with Multiple Divs
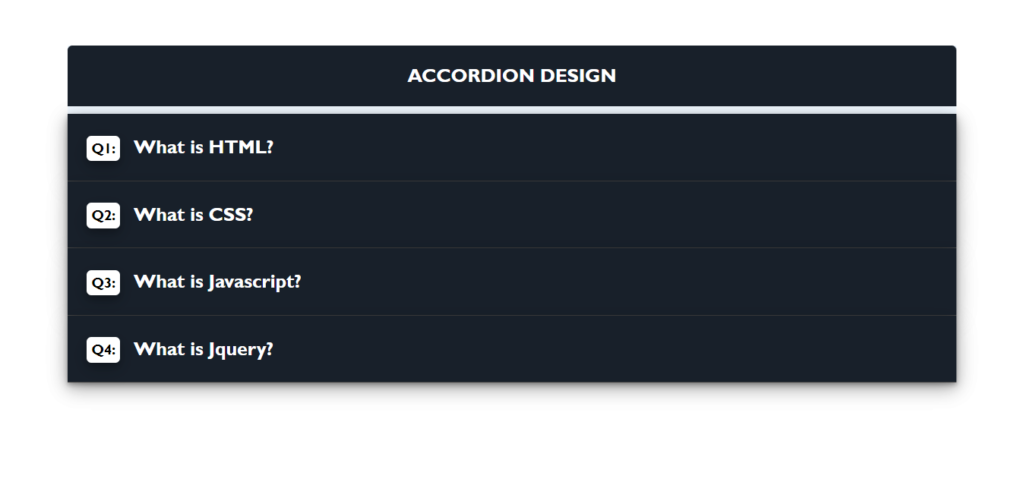
Wrap Up the JQuery Accordion with Multiple Divs
Implementing a Jquery Accordion with multiple divs is a practical way to enhance the experience of the users. By following this step-by-step guide, we also break down each line of code to ensure you understand it effortlessly. Whether you’re designing an FAQ section or building navigation menus, implementing a jQuery accordion with multiple div classes can help keep your website organized, visually appealing, and easy to navigate.If you’re looking to create a website for your business, e-commerce store, blog, or personal portfolio, don’t hesitate to contact us.
Thank You.