Resizing images is crucial for web developers looking to optimize website performance. Large images can slow down page loading times and affect the user experience. In this post, we’ll explore how to resize images in PHP using built-in functions like imagecopyresampled()
. In PHP, two primary library types are present for image manipulation: ImageMagick and GD. This post will focus on using the GD library to resize image in php.
GD Library:
Developers tend to be more familiar with the GD library due to its widespread use. This example demonstrates how to resize any image file uploaded through an HTML form. The PHP script processes the uploaded file and resize image in php accordingly.
Resize Image in PHP
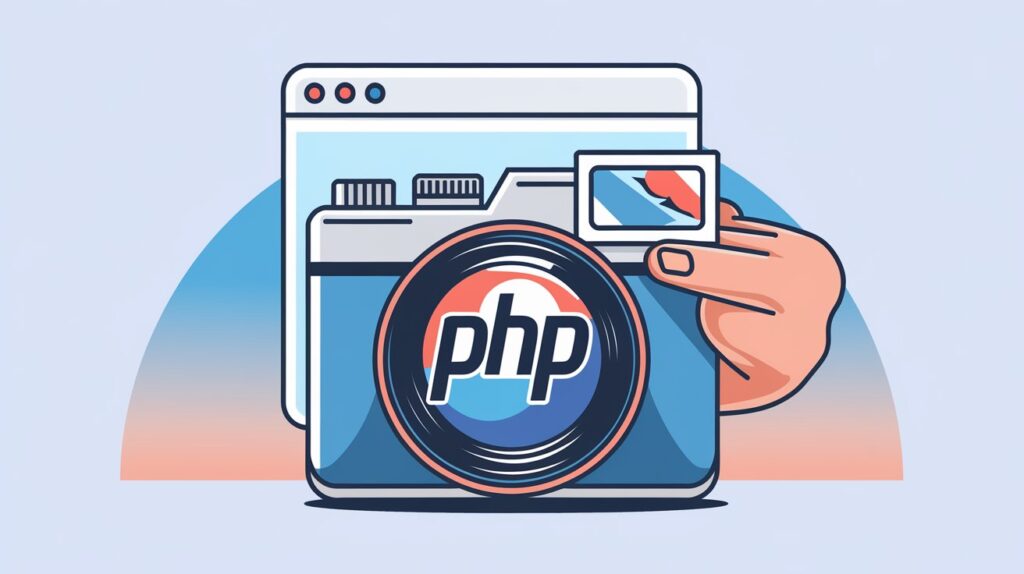
HTML and PHP code for resize image in PHP
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Resize Image in PHP</title>
<link rel="stylesheet" href="./assests/admin-css/bootstrap5.3.3.min.css">
<link rel="stylesheet" href="./assests/custom-css/image-upload.css">
<script src="./assests/admin-js/jquery3.7.1.min.js"></script>
<script src="./assests/custom-js/image-upload.js"></script>
</head>
<body>
<?php
$imgerror = $imgName = "";
if ($_SERVER['REQUEST_METHOD'] == "POST") {
$img = $_FILES['img'];
$size = $_FILES["img"]["size"];
$type = $_FILES["img"]["type"];
$name = $_FILES['img']['name'];
$tmp = $_FILES['img']['tmp_name'];
function resize($filename, int $newwidth, int $newheight)
{
$info = getimagesize($filename);
$width = $info[0];
$height = $info[1];
$mime = $info['mime'];
$image_resized = imagecreatetruecolor($newwidth, $newheight);
switch ($mime) {
case 'image/jpeg':
$image = imagecreatefromjpeg($filename);
break;
case 'image/png':
$image = imagecreatefrompng($filename);
break;
default:
return false;
}
imagecopyresampled($image_resized, $image, 0, 0, 0, 0, $newwidth, $newheight, $width, $height);
return $image_resized;
}
if ($size == 0) {
$imgerror = "Please select an image.";
} elseif ($size > 1000000) {
$imgerror = "Image size is too large.";
} elseif ($type == "image/jpeg" || $type == "image/jpg" || $type == "image/png") {
$uploadDir = './assests/images/';
if (!file_exists($uploadDir)) {
mkdir($uploadDir, 0777, true);
}
$uploadedFilePath = $uploadDir . $name;
if (move_uploaded_file($tmp, $uploadedFilePath)) {
$pathSmall = $uploadDir . 'small_' . $name;
$pathMedium = $uploadDir . 'medium_' . $name;
$pathLarge = $uploadDir . 'large_' . $name;
$new_image_small = resize($uploadedFilePath, 50, 50);
$new_image_medium = resize($uploadedFilePath, 100, 100);
$new_image_large = resize($uploadedFilePath, 200, 200);
if ($new_image_small && $new_image_medium && $new_image_large) {
imagejpeg($new_image_small, $pathSmall);
imagejpeg($new_image_medium, $pathMedium);
imagejpeg($new_image_large, $pathLarge);
$imgName = "Images uploaded successfully: " . $name;
$imgerror = "";
} else {
$imgerror = "Failed to resize the image.";
}
} else {
$imgerror = "Unable to upload the image.";
}
} else {
$imgerror = "Invalid image type. Please upload a JPEG or PNG file.";
}
}
?>
<div class="container">
<div class="image-upload-wrapper">
<h1>Upload Your Image</h1>
<form id="uploadForm" method="POST" enctype="multipart/form-data">
<div class="upload-area">
<input type="file" id="img" name="img" accept="image/*" onchange="previewImage(event)" />
<label for="img">Choose Image</label>
</div>
<div id="image-preview" class="preview-area"></div>
<span class="error-message"><?php echo $imgerror; ?></span>
<span class="show-img"><?php echo $imgName; ?></span>
<div class="button-group">
<button type="submit" class="btn btn-primary">Upload</button>
<button type="button" class="btn btn-danger" onclick="removeImage()">Remove Image</button>
</div>
</form>
</div>
</div>
</body>
</html>
PHPCODE EXPLANATION: First, create a PHP file with an appropriate name, such as “image-upload.php,” and insert the provided code into that file. The image is processed to generate three different sizes: large, medium, and small. However, you can adjust this as needed for your project.
1. In the <head>
section of the HTML, several key elements are included:
<meta charset="UTF-8">
: Ensures the page uses UTF-8 character encoding.<meta name="viewport" content="width=device-width, initial-scale=1.0">
: Makes the page responsive, adjusting to different screen sizes.<title>
: Specifies the page’s title as “Image Upload.”<link rel="stylesheet" href="./assets/admin-css/bootstrap5.3.3.min.css">
: Links to the Bootstrap CSS for styling. If you haven’t downloaded the Bootstrap file, you can use the Bootstrap CDN instead. However, to ensure proper styling, it’s mandatory to include this in your<head>
section. Don’t forget to add the CDN link.<link rel="stylesheet" href="./assets/custom-css/image-upload.css">
: Links to custom CSS for additional design.<script src="./assets/admin-js/jquery3.7.1.min.js"></script>
: Includes jQuery for dynamic functionality.If you haven’t downloaded the jQuery file, you can use the jQuery CDN instead. However, to ensure proper styling, it’s mandatory to include this in your<head>
section. Don’t forget to add the CDN link.<script src="./assets/custom-js/image-upload.js"></script>
: Links to custom JavaScript for handling the image upload process.
2. Container Section:
The container section holds the input field where users can upload an image.
Don’t forget to include enctype="multipart/form-data"
In your form tag, ensure the image is uploaded correctly. Omitting this attribute can result in a failed upload. In the input field, I’ve used the onchange
event to allow users to preview the image as soon as it’s selected. Once an image is selected, it will be shown to the user for verification.
PHP echo
Statements are used to display error messages ($imgerror
) and ($imageName) is used to display the name of the uploaded image. It button-group
includes a “Upload” button for submitting the form and a “Remove Image” button with a JavaScript function to clear the image preview.
3. $_SERVER[‘REQUEST_METHOD’] == “POST” :
This condition ensures that the code inside this block only executes when the form is submitted, not when the page is initially loaded or refreshed. After checking for the POST request, it’s good practice to add validation for the uploaded image.
To verify if an input field is empty and display an error message, you can use JavaScript. If not, check if the image file size is below a specified limit, such as 1 MB (or 4 MB, depending on your requirements). If the file size exceeds the limit, show an error message.To validate that the uploaded file is indeed an image, you can check the file’s MIME type. If the file type is incorrect, display an error message. If all checks pass, proceed with further processing of the image.
4. $uploadDir = ‘./assests/images/’;: Sets the directory path where uploaded images will be stored.
5. if (!file_exists($uploadDir)) { mkdir($uploadDir, 0777, true); } checks if the upload directory exists. If you don’t, then it will help you to create a file with the specific name.
6. $uploadedFilePath = $uploadDir . $name;: This creates the full path where the uploaded file will be stored. Where, $name
is the original name of the uploaded file.
7. move_uploaded_file($tmp, $uploadedFilePath)
: moves the uploaded file to the designated path. If successful, then it will continue with the further process.
8. $pathSmall = $uploadDir . 'small_' . $name; $pathMedium = $uploadDir . 'medium_' . $name; $pathLarge = $uploadDir . 'large_' . $name;
: Defines paths for saving resized versions of the uploaded image. These paths include prefixes (small_
, medium_
, large_
) to distinguish between different sizes of the image.
9. $new_image_small = resize($uploadedFilePath, 50, 50); $new_image_medium = resize($uploadedFilePath, 100, 100); $new_image_large = resize($uploadedFilePath, 200, 200)
;: Generates three resized versions of the uploaded image. The resize()
function is used to create these versions at different dimensions: 50×50 for small, 100×100 for medium, and 200×200 for large. You can customize these dimensions based on your specific needs.
10. After invoking the resize() function, this section of the code performs the image resizing process:
$info = getimagesize($filename);
$width = $info[0];
$height = $info[1];
$mime = $info['mime'];
$image_resized = imagecreatetruecolor($newwidth, $newheight);
switch ($mime) {
case 'image/jpeg':
$image = imagecreatefromjpeg($filename);
break;
case 'image/png':
$image = imagecreatefrompng($filename);
break;
default:
return false;
}
imagecopyresampled($image_resized, $image, 0, 0, 0, 0, $newwidth, $newheight, $width, $height);
return $image_resized;
PHP- Retrieve Image Information:
getimagesize($filename)
will fetch the original image’s width, height, and MIME type. - Create New Image Resource:
imagecreatetruecolor($newwidth, $newheight)
initializes a new true color image resource with the new width and height. - Load the Image: Depending on the MIME type (
image/jpeg
orimage/png
), the appropriate function (imagecreatefromjpeg()
orimagecreatefrompng()
) loads the original image. - Resize the Image:
imagecopyresampled()
resizes and copies the original image into the new image resource with the specified dimensions. - Return the Resized Image: The resized image resource is returned for further processing or saving.
- Note: When working with the GD library in PHP, you might encounter errors related to
imagecreatetruecolor()
andimagecopyresampled()
, such as “Function not defined” or “call to undefined function imagetruecolor()” or issues with image quality. To resolve GD library issues, navigate to your XAMPP installation directory, then go to thephp
folder and open thephp.ini
file. Search for the line “;extension=gd
“ or something similar and remove the semicolon (;
) to uncomment it. Save the file and restart your server for the changes to take effect.
11. imagejpeg($new_image_small, $pathSmall); imagejpeg($new_image_medium, $pathMedium); imagejpeg($new_image_large, $pathLarge);
saves the resized images to their respective paths. The imagejpeg()
function is used to save the resized JPEG images ($new_image_small, $new_image_medium, $new_image_large) to the server at the specified paths ($pathSmall, $pathMedium, $pathLarge). This ensures that each version of the resized image is properly stored, allowing users to access different image sizes as required.
CSS Code For Resize Image in PHP
body {
background-color: #f7f9fc;
font-family: 'Poppins', sans-serif;
}
.container {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
.image-upload-wrapper {
background: #fff;
padding: 40px;
box-shadow: 0px 8px 20px rgba(0, 0, 0, 0.1);
border-radius: 10px;
max-width: 400px;
width: 100%;
text-align: center;
}
h1 {
font-size: 24px;
font-weight: bold;
margin-bottom: 20px;
color: #333;
}
.upload-area {
position: relative;
margin-bottom: 20px;
}
input[type="file"] {
display: none;
}
label[for="img"] {
display: inline-block;
padding: 10px 25px;
background-color: #007bff;
color: #fff;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s;
}
label[for="img"]:hover {
background-color: #0056b3;
}
.preview-area {
margin-bottom: 20px;
}
.preview-area img {
max-width: 100%;
height: auto;
border-radius: 10px;
box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1);
}
.error-message {
color: #e74c3c;
font-size: 14px;
}
.show-img {
color: #28a745;
font-size: 14px;
}
.button-group {
display: flex;
justify-content: space-between;
gap: 10px;
}
button[type="submit"], button[type="button"] {
padding: 10px 20px;
border: none;
color: #fff;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
transition: background-color 0.3s;
}
button[type="submit"] {
background-color: #28a745;
}
button[type="submit"]:hover {
background-color: #218838;
}
button[type="button"] {
background-color: #dc3545;
}
button[type="button"]:hover {
background-color: #c82333;
}
CSSCODE EXPLANATION:
- Body{..}: For the styling of the whole body, we have applied a light background color and the ‘Poppins’ font across the entire page.
- .container {..}: Centers the content vertically and horizontally within the viewport.
- .image-upload-wrapper {..}: Styles the main container with a white background, padding, shadow, rounded corners, and center-aligned text.
- h1 {..}: Sets the font size, weight, color, and margin for the heading.
- .upload-area {..}: Positions the upload area relative to its container and adds the bottom margin.
- input[type=”file”] {..} label[for=”img”] {..} label[for=”img”]:hover {…}: Hides the file input and styles the label as a clickable button with background color, padding, rounded corners, and a hover effect.
- .preview-area {..} .preview-area img {..}: Adds margin to the preview area and styles the image with rounded corners and a subtle shadow.
- .error-message {..} .show-img {..}: Colors the error message in red and the success message in green.
- .button-group {..}: Aligns buttons in a flex container with space between them.
- button[type=”submit”], button[type=”button”] { …} button[type=”submit”] {…} button[type=”submit”]:hover {…} button[type=”button”] {…} button[type=”button”]:hover {…}: Styles submit and button elements with padding, border-radius, font size, and transition effects. Different background colors and hover states are applied to the submit and cancel buttons.
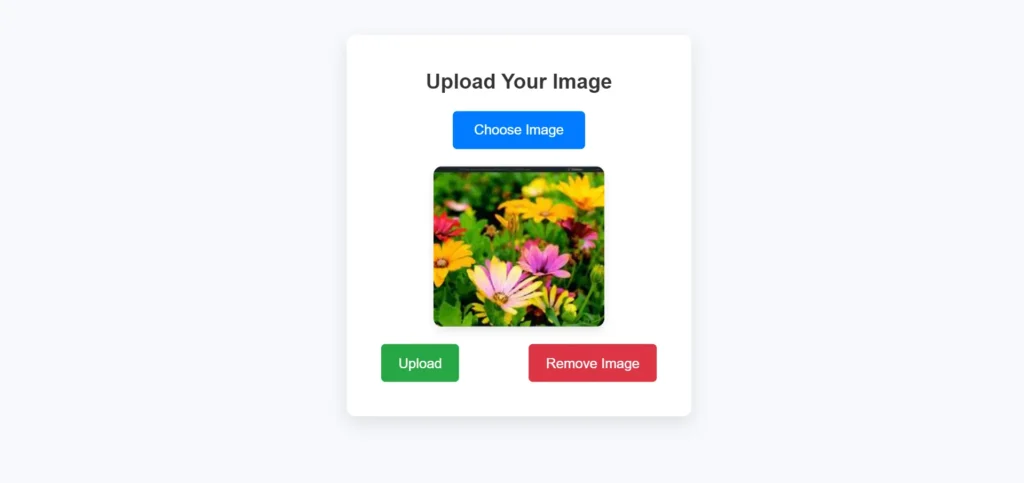
JQuery CODE for Resize Image in PHP
function previewImage(event) {
var reader = new FileReader();
reader.onload = function() {
$('#image-preview').html('<img src="' + reader.result + '" alt="Image Preview" class="img-fluid" />');
}
reader.readAsDataURL(event.target.files[0]);
}
function removeImage() {
$('#img').val('');
$('#image-preview').html('');
}
JQueryCODE EXPLANATION:
- Preview Image: This function uses the
FileReader
API to read the selected image file and display it as a preview. When a user selects an image, thereader.onload
the function sets the image source to the file’s data URL, which is then displayed in the#image-preview
div. - Remove Image: This function clears the selected file and removes the preview image. It resets the file input and empties the
#image-preview
div, allowing users to select a new image or cancel the upload.
Output
Here is the output video of the above code:
Output Images:
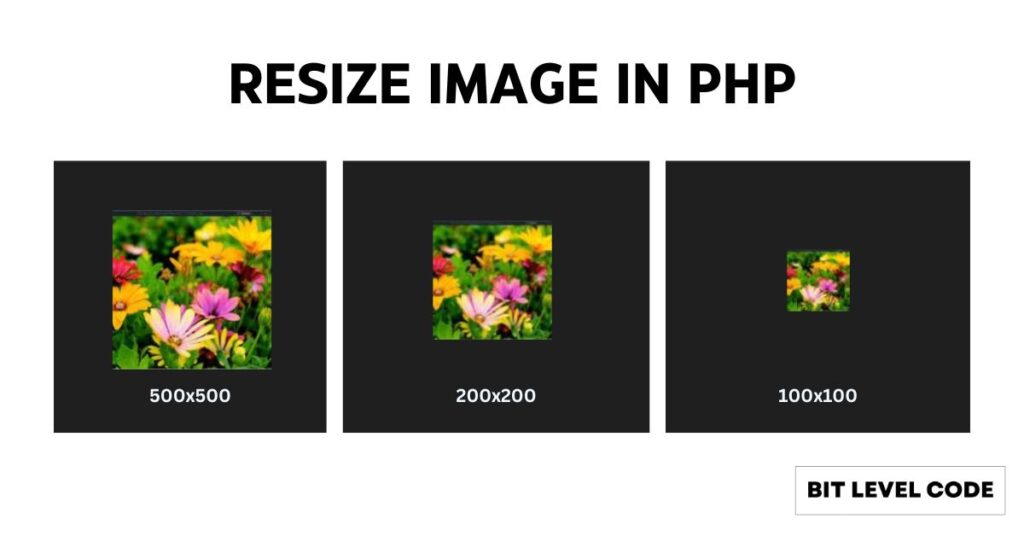
Related Post:
>> 5 Ways to Sort Array in PHP Without Using Inbuilt Function
>> How to Create JQuery Accordion with Multiple Divs
Conclusion for Resize image in PHP
Resize image in PHP, CSS, and jQuery might seem intricate at first, but with the right approach, it becomes manageable and effective. By utilizing PHP’s GD library for image manipulation, you can resize image in PHP to various dimensions to fit different needs. CSS styles enhance the visual presentation of the upload form, ensuring a user-friendly interface, while jQuery facilitates real-time image previews and interactive features.
Mastering these techniques not only improves your web development skills but also allows you to create more dynamic and responsive web applications. Whether you’re refining the user experience, optimizing image handling, or simply exploring new technologies, these practices are essential for modern web development.
If you have any questions or need further assistance with resize image in PHP , CSS styling, or jQuery functionality, feel free to reach out!
Happy Coding!