Looking for a way to convert Word files to PDF in PHP? This guide covers everything you need to know to achieve this using Dompdf. Learn the step-by-step process with easy-to-follow code examples to seamlessly convert Word documents into PDF files. This is perfect for developers aiming to simplify document sharing and enhance user experience.
Table of Contents
How to Convert Word Files to PDF in PHP: Install Dompdf and PHPWord Using Composer
If you’re looking to convert Word files to PDF in PHP, the process becomes much easier when you use libraries like Dompdf and PHPWord. In this guide, we will walk you through the installation process of these two essential libraries, using Composer — a dependency management tool for PHP.
Step 1: Install Composer
Before you can install Dompdf and PHPWord, you need to have Composer installed on your system. Composer allows you to manage dependencies for your PHP projects and makes the installation process seamless.
Steps to Install Composer:
- Visit Composer’s official website to download the latest version.
- Follow the instructions for your operating system:
- For Windows: Download the Composer Setup installer and run it.
- For macOS/Linux: Open the terminal and follow the command-line installation instructions.
If you don’t have Composer installed, you can follow this detailed guide to install Composer on Windows from GeeksforGeeks.
Once installed, verify Composer is working by running:
composer --version
PHPIf installed correctly, this will display the Composer version.
Step 2: Navigate to Your Project Folder
If you are using XAMPP or WAMP, you need to navigate to the folder where your PHP project is located.
- If you’re using XAMPP, run the following command to navigate to your project folder.
cd C:\xampp\htdocs\php_project
PHP- if you’re using WAMP, run the following command:
cd C:\wamp64\www\php_project
PHPAfter navigating to your project directory, you can install Dompdf and PHPWord.
Step 3: Install Dompdf and PHPWord
Now that you have Composer installed and you’re in the correct directory, it’s time to install the libraries required to convert Word files to PDF in PHP.
Steps to Install Dompdf:
- Open your terminal (or command prompt) in your project directory.
- Run the following command to install Dompdf.
composer require dompdf/dompdf
PHPDompdf is a popular library used to generate PDF files from HTML content. It will allow you to create PDF files easily once the Word file is converted to HTML
Steps to Install PHPWord:
- In the same terminal window, run the following command to install PHPWord.
composer require phpoffice/phpword
PHP- PHPWord is an open-source library that helps you read and write Word documents in PHP. You’ll use this to load and process Word files before converting them into PDF.
Step 4: Use Dompdf and PHPWord to Convert Word Files to PDF
Now that both libraries are installed, you can begin the process of converting Word files to PDF.
Why You Should Install Dompdf and PHPWord
- Dompdf: This library is essential for generating high-quality PDFs from HTML content. Once you convert a Word document into HTML, Dompdf allows you to render it into a professional PDF format that can be shared, printed, or archived.
- PHPWord: This library makes it possible to read and manipulate Word documents programmatically. PHPWord handles various Word document formats (DOCX, DOC), enabling you to extract the content and prepare it for conversion into a PDF.
Step-by-Step Guide to Convert Word Files to PDF in PHP: PHP Code for Uploading and Conversion
To convert Word files to PDF in PHP, you need two essential files: one for uploading the Word file and another for processing and converting the file into PDF. Below are the two PHP files required for this process:
- pdf.php: This file will handle the Word file upload.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Upload DOCX</title>
<style>
body {
font-family: Arial, sans-serif;
background: linear-gradient(120deg, #f0f8ff, #e6e6fa);
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.upload-container {
background: white;
padding: 30px;
border-radius: 10px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
text-align: center;
max-width: 400px;
width: 100%;
}
.upload-container h1 {
font-size: 24px;
color: #333;
margin-bottom: 20px;
}
.upload-container input[type="file"] {
margin: 20px 0;
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
font-size: 16px;
width: 100%;
cursor: pointer;
}
.upload-container button {
background-color: #4caf50;
color: white;
font-size: 18px;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
.upload-container button:hover {
background-color: #45a049;
}
.footer {
margin-top: 20px;
font-size: 14px;
color: #555;
}
</style>
</head>
<body>
<div class="upload-container">
<h1>Convert DOCX to PDF</h1>
<form action="pdf_output.php" method="post" enctype="multipart/form-data">
<input type="file" name="docx_file" accept=".docx" required>
<button type="submit">Upload and Convert</button>
</form>
<p class="footer">Supported file format: DOCX</p>
</div>
</body>
</html>
PHP- pdf_output.php: This file will process the uploaded Word file and convert it to a PDF.
<?php
require 'vendor/autoload.php';
use PhpOffice\PhpWord\IOFactory;
use Dompdf\Dompdf;
use Dompdf\Options;
// Check if a file was uploaded
if ($_SERVER['REQUEST_METHOD'] === 'POST' && isset($_FILES['docx_file'])) {
$fileTmpPath = $_FILES['docx_file']['tmp_name'];
$fileName = $_FILES['docx_file']['name'];
// Validate file type
$fileType = pathinfo($fileName, PATHINFO_EXTENSION);
if ($fileType !== 'docx') {
die('Error: Please upload a DOCX file.');
}
// Load the DOCX file
$phpWord = IOFactory::load($fileTmpPath);
// Convert the DOCX to HTML
$htmlWriter = \PhpOffice\PhpWord\IOFactory::createWriter($phpWord, 'HTML');
ob_start();
$htmlWriter->save('php://output');
$htmlContent = ob_get_clean();
// Initialize Dompdf
$options = new Options();
$options->set('defaultFont', 'Arial');
$dompdf = new Dompdf($options);
// Load HTML content to Dompdf
$dompdf->loadHtml($htmlContent);
// Set paper size and orientation
$dompdf->setPaper('A4', 'portrait');
// Render the PDF
$dompdf->render();
// Output the PDF to browser
$dompdf->stream('converted.pdf', ['Attachment' => true]);
} else {
die('No file uploaded.');
}
PHPOutput: Convert Word Files to PDF in PHP
Before Uploading The File:
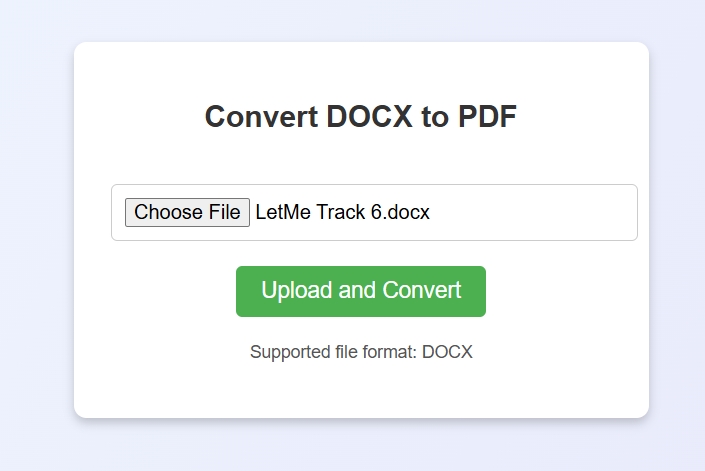
After Uploading The File:

Related Posts:
>>How to Send Email from Localhost in PHP Using PHPMailer
>>How to Create a WordPress Theme: 2 Easy Steps
Conclusion: Convert Word Files to PDF in PHP
By following the steps outlined in this guide, you can easily convert Word files to PDF in PHP before uploading them, using the powerful Dompdf and PHPWord libraries. With these tools, your PHP projects will be able to handle Word document conversions seamlessly, ensuring a smoother workflow and improved functionality.
Now that you’ve learned how to set everything up, you’re ready to implement this feature into your projects!
Happy coding! 🚀